文章目录[x]
- 0.1:一、SQLSugar功能介绍
- 0.2:二、 .NetFramework中使用sqlsugar
之前在做坦克游戏的服务端的时候就打算引入SqlSugar,但是由于时间的关系没有实现。为什么要使用sqlsugar这样一个ORM框架?因为使用ORM框架可以提高开发效率,降低开发成本 、使开发更加对象化 、方便移植 等优点。在此之前还尝试过使用EntityFramework,可能是因为自己对这块不熟悉花了半天时间才连接成功,感觉EF还是搭配sqlserver更合适。
一、SQLSugar功能介绍
1. 相比EF Core,多库支持更好 ,在国内拥有不逊色EF Core 的开源生态;
2. 支持 .NET 百万级【大数据】写入和更新、分表和几十亿查询和统计等 拥有成熟方案;
3. 支持 完整的SAAS一套应用 跨库查询 、租户分库 、租户分表 和 租户数据隔离;
4. 支持【低代码】+工作流 (无实体多库兼容CRUD & JSON TO SQL );
5. 语法最爽的ORM、优美的表达式、仓储、UnitOfWork、DbContext、AOP ;
6. 支持 DbFirst、CodeFirst和【WebFirst】 3种模式开发;
7. 简单易用、功能齐全、高性能、轻量级、服务齐全、官网教程文档、有专业技术支持一天18小时服务。
官方网站:https://www.donet5.com/Home/Doc
二、 .NetFramework中使用sqlsugar
1.新建解决方案,自定义解决方案名称和保存路径。
2.在项目中添加相关程序包(mysql.data和sqlsugar),安装mysql.data的时候会自动安装依赖包google.protobuf。
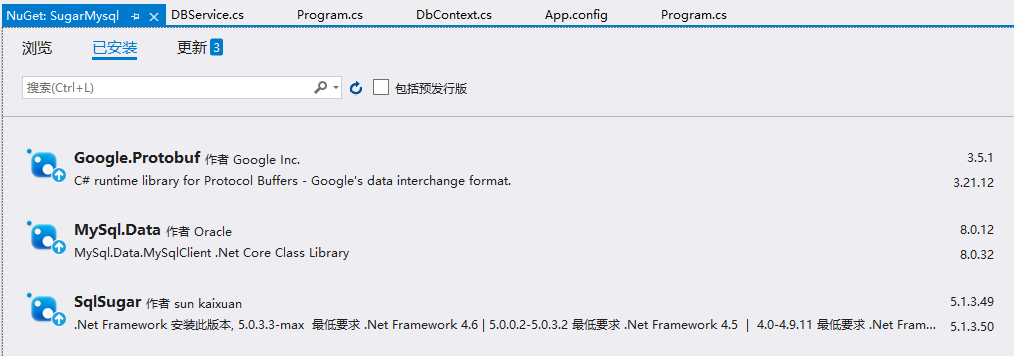
3.封装一个数据库操作类。
using SqlSugar; using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; namespace SugarMysql { public class DBContext<T> where T : class, new() { public SqlSugarClient Db; private static DBContext<T> mSingle = null; public static DBContext<T> GetInstance() { if (mSingle == null) mSingle = new DBContext<T>(); return mSingle; } protected DBContext() { //通过这个可以直接连接数据库 Db = new SqlSugarClient(new ConnectionConfig() { //可以在连接字符串中设置连接池pooling=true;表示开启连接池 //eg:min pool size=2;max poll size=4;表示最小连接池为2,最大连接池是4;默认是100 ConnectionString = "database='" + "sugarMysql" + "';Data Source = '" + "172.30.146.184" + "'; User Id = '" + "root" + "'; pwd='" + "123456" + "';charset='utf8mb4';pooling=true", DbType = SqlSugar.DbType.MySql,//我这里使用的是Mysql数据库 IsAutoCloseConnection = true,//自动关闭连接 InitKeyType = InitKeyType.Attribute }); } public void Dispose() { if (Db != null) { Db.Dispose(); } } public SimpleClient<T> CurrentDb { get { return new SimpleClient<T>(Db); } } /// <summary> /// 获取所有 /// </summary> /// <returns></returns> public virtual List<T> GetList() { return CurrentDb.GetList(); } /// <summary> /// 根据表达式查询 /// </summary> /// <returns></returns> public virtual List<T> GetList(Expression<Func<T, bool>> whereExpression) { return CurrentDb.GetList(whereExpression); } /// <summary> /// 根据主键查询 /// </summary> /// <returns></returns> public virtual List<T> GetById(dynamic id) { return CurrentDb.GetById(id); } /// <summary> /// 根据主键删除 /// </summary> /// <param name="id"></param> /// <returns></returns> public virtual bool Delete(dynamic id) { if (string.IsNullOrEmpty(id.ObjToString)) { Console.WriteLine(string.Format("要删除的主键id不能为空值!")); } return CurrentDb.Delete(id); } /// <summary> /// 根据实体删除 /// </summary> /// <param name="id"></param> /// <returns></returns> public virtual bool Delete(T data) { if (data == null) { Console.WriteLine(string.Format("要删除的实体对象不能为空值!")); } return CurrentDb.Delete(data); } /// <summary> /// 根据主键批量删除 /// </summary> /// <param name="id"></param> /// <returns></returns> public virtual bool Delete(dynamic[] ids) { if (ids.Count() <= 0) { Console.WriteLine(string.Format("要删除的主键ids不能为空值!")); } return CurrentDb.AsDeleteable().In(ids).ExecuteCommand() > 0; } /// <summary> /// 根据表达式删除 /// </summary> /// <param name="id"></param> /// <returns></returns> public virtual bool Delete(Expression<Func<T, bool>> whereExpression) { return CurrentDb.Delete(whereExpression); } /// <summary> /// 根据实体更新,实体需要有主键 /// </summary> /// <param name="id"></param> /// <returns></returns> public virtual bool Update(T obj) { if (obj == null) { Console.WriteLine(string.Format("要更新的实体不能为空,必须带上主键!")); } return CurrentDb.Update(obj); } /// <summary> ///批量更新 /// </summary> /// <param name="id"></param> /// <returns></returns> public virtual bool Update(List<T> objs) { if (objs.Count <= 0) { Console.WriteLine(string.Format("要批量更新的实体不能为空,必须带上主键!")); } return CurrentDb.UpdateRange(objs); } /// <summary> /// 插入 /// </summary> /// <param name="id"></param> /// <returns></returns> public virtual bool Insert(T obj) { return CurrentDb.Insert(obj); } /// <summary> /// 批量 /// </summary> /// <param name="id"></param> /// <returns></returns> public virtual bool Insert(List<T> objs) { return CurrentDb.InsertRange(objs); } //可以扩展更多方法 } }
4.定义数据model类。
[SugarTable("Books")]//指定数据库中的表名,要对应数据库的表名,否则会出错 public class Books { [SugarColumn(IsPrimaryKey = true, IsIdentity = true)]//指定主键和自动增长 public int Id { get; set; } public int BId { get; set; } public string BName { get; set; } public int TypeId { get; set; } }
5.操作数据库。
static void Main(string[] args) { //下面代码只是演示CRUD,数据库里没有数据的情况下下面代码会报错 Books b = new Books() { BId = 2, BName = "MySQL", TypeId = 5 }; //添加数据 DBContext<Books>.GetInstance().CurrentDb.Insert(b); //删除数据 DBContext<Books>.GetInstance().CurrentDb.Delete(b); //更新数据 DBContext<Books>.GetInstance().CurrentDb.Update(b); //插找数据 DBContext<Books>.GetInstance().CurrentDb.GetById(0); }